Interesting C Programming Tricks You didn’t know before
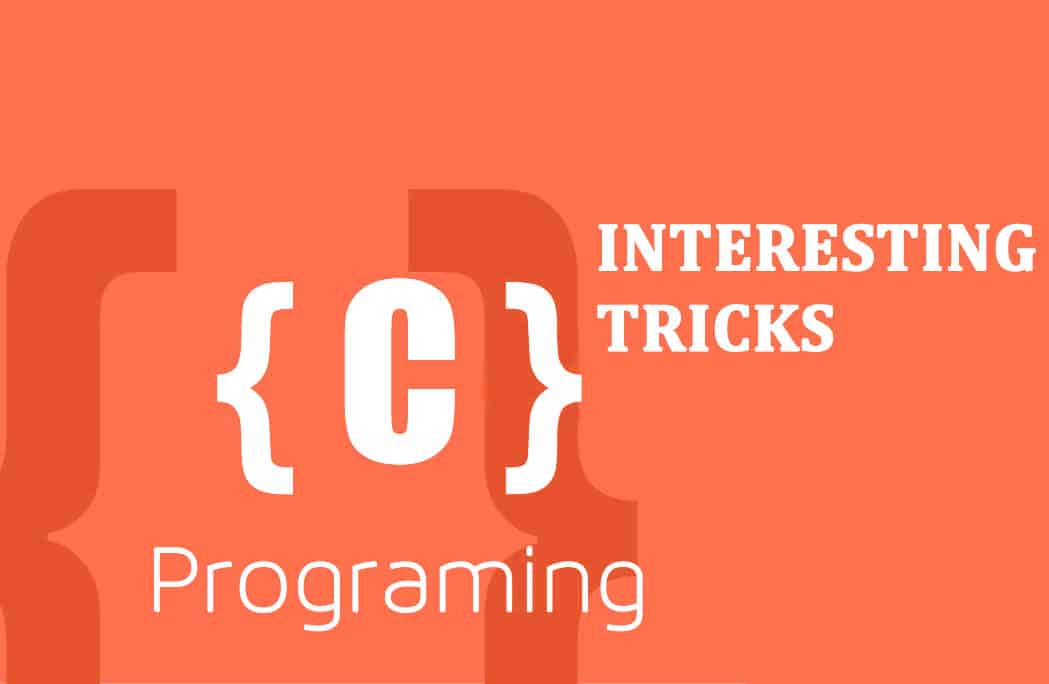
Top 10 Interesting C programming tricks
1. Using the return value of “scanf()” to check for end of file:
while(~scanf(“%d”,&n)) {
/* Your solution */
}
Very useful at online judges where inputs are terminated by EOF.
2. “%m” when used within printf() prints “Success”:
printf(“%m”);
%m only prints “Success” when “errno == 0”, it’s short for a string representation of the last observed error state. For example if a function fails before the printf then it will print something rather different. *
3. Implicit initialization of integer variables with 0 and 1.
main(i){
printf(“g = %d, i = %d \n”,g,i);
}
4. brk(0); can be used as an alternative for return 0;:
5. Print Numbers 1 to 200 wihout using any loop, goto, recursion
#include<stdio.h>
#define STEP1 step();
#define STEP2 STEP1 STEP1
#define STEP4 STEP2 STEP2
#define STEP8 STEP4 STEP4
#define STEP16 STEP8 STEP8
#define STEP32 STEP16 STEP16
#define STEP64 STEP32 STEP32
#define STEP128 STEP64 STEP64
#define STEP256 STEP128 STEP128
int n = 0;
int step()
{
if (++n <= 200)
printf(“%d\n”, n);
}
int main()
{
STEP256;
return 1;
}
7. C++ Sucks?
#include <stdio.h>
double m[]= {7709179928849219.0, 771};
int main()
{
m[1]–?m[0]*=2,main():printf((char*)m);
}
You will be amazed to see the output: C++Sucks
8. Do you know “GOES TO-->” operator in C?
Actually –> is not an operator. In fact, it’s a combination of two separate operators i.e. — and >. To understand how “goes to” operator works, go through the below code snippet.
In the example, there is conditional’s code which decrements variable x, while returning x’s original (not decremented) value, and then compares the original value with 0 using the > operator.
int _tmain(){
int x = 10;
while( x –> 0 ) // x goes to 0
{
printf(“%d “, x);
}
printf(“\n”);
}
Output:
9 8 7 6 5 4 3 2 1 0
Press any key to continue . . .
9. Scanf Magic
scanf(“%[^,]”, a); // This doesn’t scrap the comma
scanf(“%[^,],”,a); // This one scraps the comma
scanf(“%[^\n]\n”, a); // It will read until you meet ‘\n’, then trashes the ‘\n’
scanf(“%*s %s”, last_name); // last_name is a variable
10. Add numbers without ‘+’ operator
int Add(int x, int y)source: technotification
{
if (y == 0)
return x;
else
return Add( x ^ y, (x & y) << 1);
}